PayFlow recurring billing with ActiveMerchant
30 Oct 2009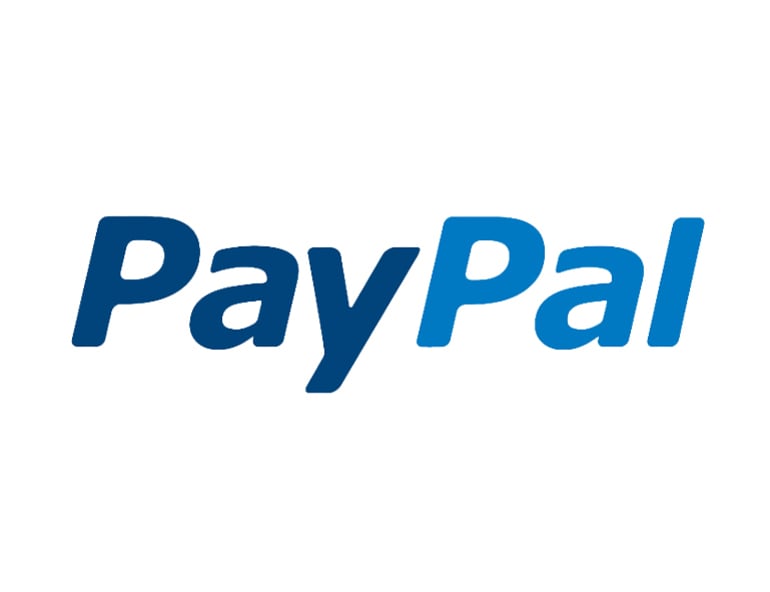
Today we are going to look at using ActiveMerchant to set up a recurring billing subscription with PayFlow .
PayFlow is Paypal's payment gateway and you need to setup a PayFlow account.
IMPORTANT! This is separate from Paypal's development sandbox. Follow these steps to setup a Payflow testing account
- Go to https://www.paypal.com/cgi-bin/webscr?cmd=_payflow-get-started-outside
and fill in the details for an account. - When you get to the page where you need to enter your payment information, hit Save and Exit. This will create a testing PayFlow account for you.
- You will be sent an email with your partner ID and your vendor login. Take note of your partner ID as this will be important later.
- You should now be able to login at https://manager.paypal.com/
Now that you have a PayFlow account, you can use ActiveMerchant to setup payments. For now we will muck around in irb to test that methods out.
So lets open up irb and start by including the active merchant gem and setting ActiveMerchant to test mode
kongy@Deadpool: $ irb
irb(main):001:0> require 'rubygems'
irb(main):002:0> require 'active_merchant'
irb(main):003:0> ActiveMerchant::Billing::Base.mode = :test
Now lets setup the gateway.
gateway = ActiveMerchant::Billing::PayflowGateway.new(:login => 'PAYFLOW_LOGIN', :password => 'PAYFLOW_PASSWORD', :partner => 'PARTNER_ID')
This creates the gateway that we will be using to request purchases. By default ActiveMerchant passes PAYPAL as the partner value if you leave it out. I believe that this is the default for US PayFlow account. For my Aussie one, I received a VSA partner_id. I would suggest putting it in there anyway.
PayFlow Testing only accepts testing credit cards numbers. You can grab them from the PayFlow recurring billing documentation found here. Here is a quick list which I can't guarantee will be up to date.
- American Express
- 378282246310005
- American Express
- 371449635398431
- American Express Corporate
- 378734493671000
- Diners Club
- 30569309025904
- Diners Club
- 38520000023237
- Discover
- 6011111111111117
- Discover
- 6011000990139424
- JCB
- 3530111333300000
- JCB
- 3566002020360505
- MasterCard
- 5555555555554444
- MasterCard
- 5105105105105100
- Visa
- 4111111111111111
- Visa
- 4012888888881881
- Visa
- 4222222222222
So lets create a Mastercard credit card.
irb(main):004:0> credit_card = ActiveMerchant::Billing::CreditCard.new( :number => '5105105105105100', :month => '9', :year => '2007', :first_name => 'Mal', :last_name => 'Reynolds', :verification_value => '123', :type => 'master' )
Now we are ready to start billing. If you want to setup a one time payment it is quite easy.
irb(main):007:0> response = gateway.purchase(1000, credit_card)
irb(main):008:0> response.success?
=> true
If you go into your Paypal Manager and search for transactions you should see it appear.
To setup a recurring billing we need to use the recurring method of the gateway. The recurring method accepts the amount in cents, the credit card object and the time intervals to charge the card, at a minimum. There are other options available which you can find here. Lets charge $10/month
irb(main):009:0> response = gateway.recurring(100, credit_card, :periodicity => :monthly)
irb(main):010:0> response.success?
=> true
irb(main):011:0> response.profile_id
=> "RT0000000002"
You can view the recurring billings in your Paypal Manager by clicking on Service Settings > Recurring Billings > Manage Profiles. You will probably want to store the profile_id in your database for when you need to edit details of the recurring billing. You can do it quite simply by calling the recurring method again. Let's change the amount we want to bill to $20/week.
irb(main):0012:0> response = gateway.recurring(2000, nil, :profile_id => "RT0000000001", :periodicity => :weekly)
irb(main):013:0> response.success?
=> true
You can see here that we no longer need to pass in the credit card since we have the profile_id. We update the amount, and change the periodicity of the billing.
And that's it.